Deploy to Vercel
Deploying JStack to Vercel is like deploying any other Next.js app, it works out of the box. This guide will walk you through the deployment process.
Deployment Steps
-
Configure Client URL
Update
lib/client.ts
to use the Vercel URL in production. Vercel automatically provides your deployment URL asprocess.env.VERCEL_URL
:lib/client.ts
-
Deploy to Vercel
- Via GitHub: Connect repository through Vercel dashboard
- Via CLI: Run
vercel deploy
- Vercel automatically sets the production URL
Environment Variables
Configure your environment variables in the Vercel dashboard:
- Go to your project settings
- Navigate to the "Environment Variables" tab
- Add your variables
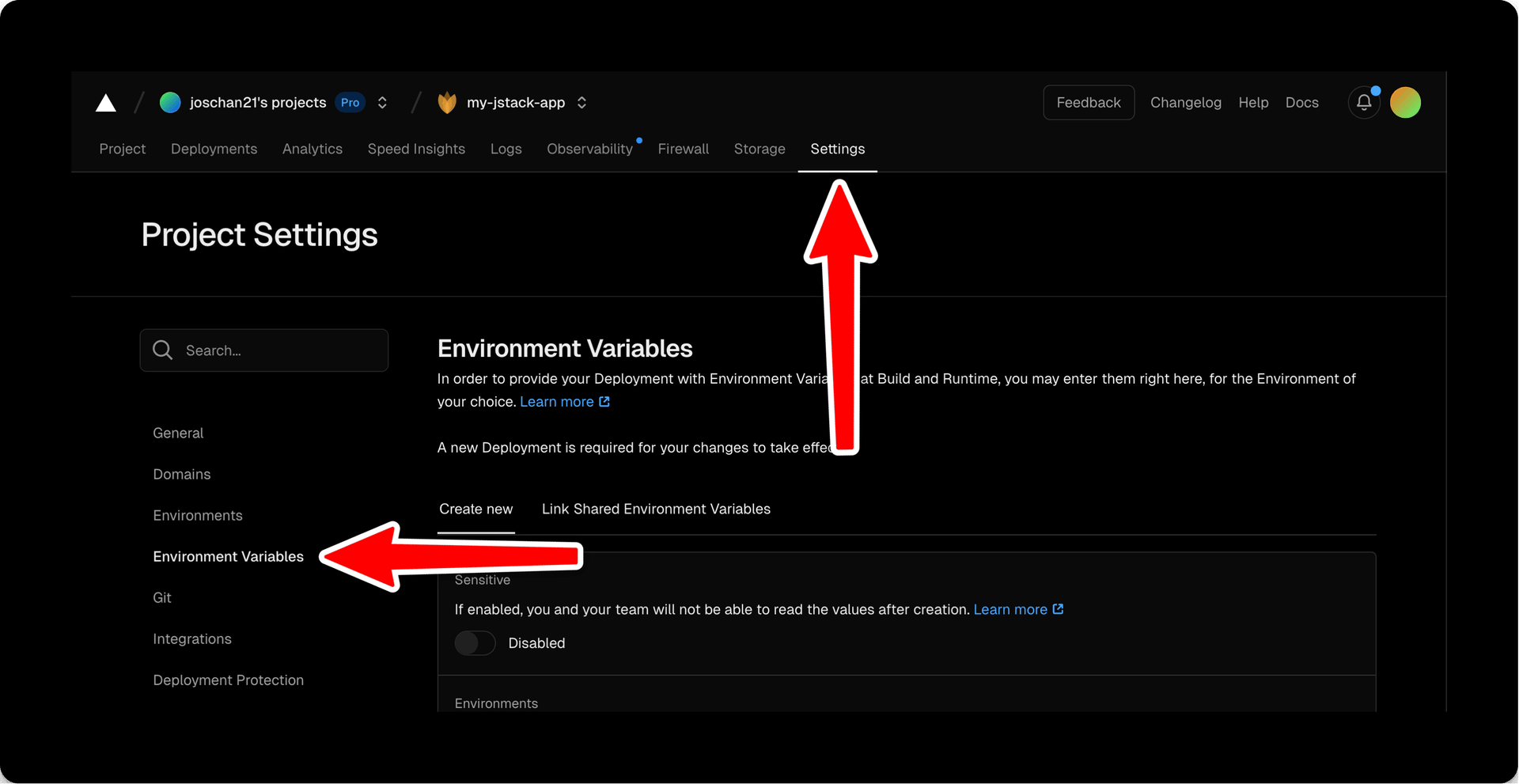
Alternatively, you can use the CLI to add environment variables:
Terminal
Common Problems
CORS Configuration
If you're experiencing CORS problems, make sure your base API includes CORS middleware:
server/index.ts